Android 15 has introduced a variety of powerful features that bring new opportunities for developers. From improved performance optimizations to advanced APIs and increased focus on security and privacy, this release is packed with new tools for creating better apps. In this article, we’ll dive into these features with code snippets to help you quickly adapt your projects to Android 15.
Performance Optimizations with Memory Management
One of the key highlights in Android 15 is its focus on efficient memory and CPU usage. Developers can now take advantage of Android’s enhanced Memory Management API to optimize the memory consumption of their apps. Here’s a quick example of how to monitor memory usage using the Debug
class:
val usedMemory = Debug.getNativeHeapAllocatedSize() / 1024
Log.d("MemoryUsage", "App is using $usedMemory KB of native heap memory.")
By periodically checking memory usage, you can optimize resource-heavy tasks and ensure your app runs smoothly, especially on lower-end devices.
Security and Privacy: Enhanced Permissions Management
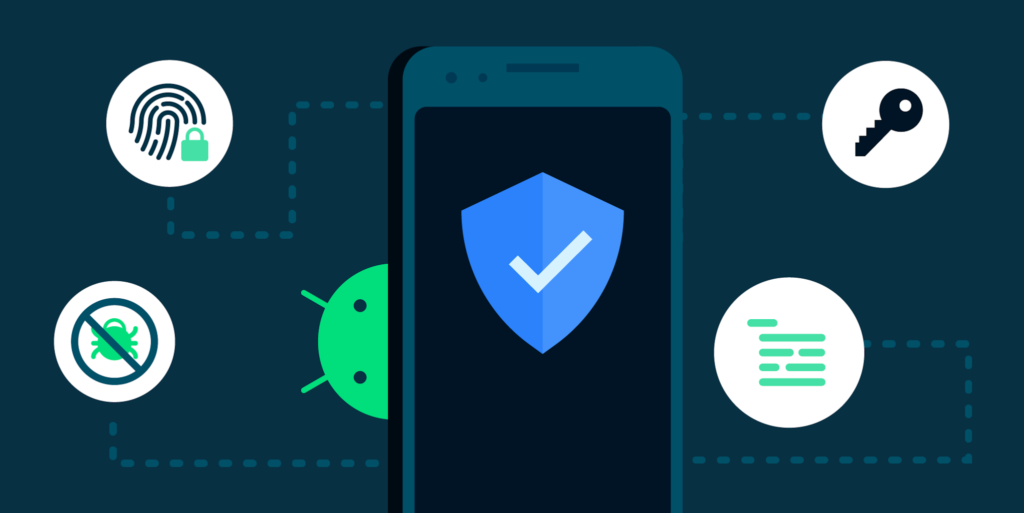
Security has been a major focus in Android 15, with improved permissions management. The updated runtime permission system allows users to control app access to sensitive data more efficiently. As a developer, you need to ensure your app requests permissions at the right time. Below is an example of requesting camera permission:
if (ContextCompat.checkSelfPermission(this, Manifest.permission.CAMERA)
!= PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(this, arrayOf(Manifest.permission.CAMERA), REQUEST_CODE_CAMERA)
} else {
// Permission already granted
openCamera()
}
Make sure to handle permission responses properly and follow best practices to enhance security in your apps.
AI and Machine Learning: Leveraging Neural Networks API
Android 15 enhances support for Neural Networks API (NNAPI), allowing developers to integrate advanced AI and ML models more easily. This can be particularly useful for features like image classification and real-time object detection. Here’s an example of how to set up NNAPI for inference:
val nnApiOptions = Interpreter.Options().apply {
setUseNNAPI(true)
}
val tfliteInterpreter = Interpreter(modelBuffer, nnApiOptions)
// Run inference on the model
tfliteInterpreter.run(inputData, outputData)
This small snippet demonstrates the power of NNAPI for running machine learning models efficiently on Android 15 devices, allowing for faster inferences and improved AI-driven features.
Material You: Dynamic Theming in Android 15
Android 15’s Material You design language brings a highly customizable, user-centric experience. Developers can now create apps that adapt to users’ chosen color palettes dynamically. To implement dynamic theming, you can retrieve system colors and apply them across your UI components:
val primaryColor = MaterialColors.getColor(context, R.attr.colorPrimary, "Primary color not found")
view.setBackgroundColor(primaryColor)
This simple yet powerful feature enables your app to seamlessly blend with the user’s preferred theme, providing a cohesive experience.
Game Mode API for Better Gaming Performance
Android 15 introduces the Game Mode API, which allows developers to optimize their games for different modes, such as performance or battery-saving modes. By utilizing the GameModeManager
, you can tailor your game’s behavior based on user preferences:
val gameModeManager = getSystemService(GameModeManager::class.java)
gameModeManager.setGameMode(GameModeManager.MODE_PERFORMANCE)
// Adjust game settings for optimal performance
This feature ensures that your game takes full advantage of the system’s resources when users prioritize performance, or conserves battery when needed.
Conclusion: Embracing the Future with Android 15
Android 15 brings a host of exciting features that help developers create more efficient, secure, and personalized apps. From the improved memory management to the enhanced permissions system and powerful AI integrations, the potential is limitless. By leveraging these features, you can optimize your apps and provide users with a superior experience.
Source: Android 15 Developer Guide