Hey developers! 👋 Today, we’re diving deep into how you can retrieve cookies from a WebView in Android using Kotlin. Whether you need session data or any other crucial information for your app, understanding how to properly manage and fetch cookies is vital for improving user experience. Let’s get started and explore everything step by step!
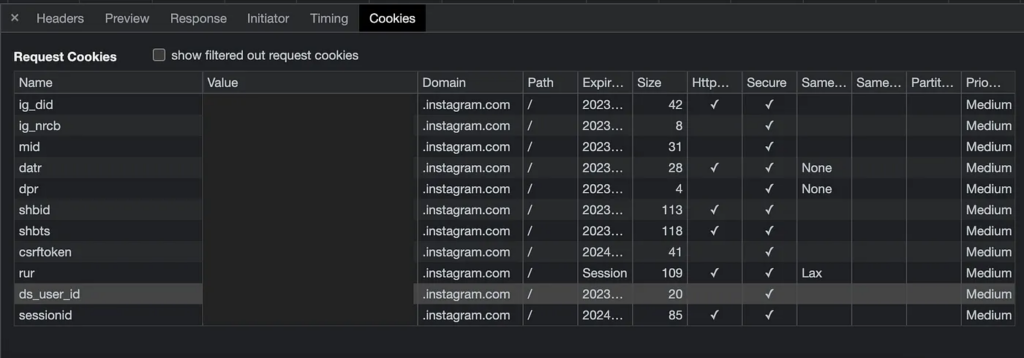
Why Retrieve Cookies in a WebView?
Before we dive into the technical side, let’s first clarify why you’d want to retrieve cookies from a WebView. In most web applications, cookies are used to store small amounts of data. These could include:
- Session Data: Allows users to remain logged in without re-entering credentials.
- User Preferences: Stores settings such as language, themes, etc.
- Authentication Tokens: Useful for maintaining secure, persistent login sessions.
For mobile apps that embed web pages using WebView, accessing cookies can help sync web and app sessions. For example, if your app involves logging in via a web page and you want to maintain that session in your app, you’ll need to interact with cookies. This brings us to our next point — how to set up a WebView.
Setting Up the WebView Component
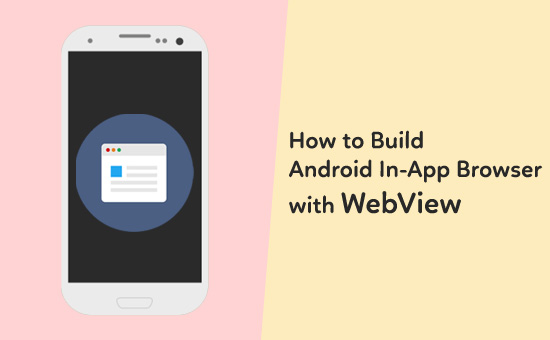
First things first, you’ll need to add a WebView to your app’s layout. Here’s how to define it in the activity_main.xml
layout file:
<WebView
android:id="@+id/webView"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
Now that we have our WebView defined, let’s load a URL into it. In this case, we’ll use Instagram’s login page as an example. This will load the web page inside the WebView.
binding.webView.loadUrl("https://www.instagram.com/accounts/login/")
Why Instagram? Well, Instagram’s login page is a typical example where cookies store session data, and it’s a good test case for understanding how WebView works with cookies.
Creating a Custom WebViewClient
By default, when a user interacts with links inside a WebView, the system opens those links in the default browser, which can interrupt the user experience. To manage navigation within the WebView itself, we need to create a custom WebViewClient
. This allows us to handle page navigation and track when the page loading finishes.
class MyWebViewClient : WebViewClient() {
// This function is called when a page finishes loading in the WebView
override fun onPageFinished(view: WebView?, url: String?) {
super.onPageFinished(view, url)
// Check if we've reached the desired page
url?.let {
if (it.contains("instagram.com")) {
// Handle cookies here after the page is fully loaded
retrieveCookies(url)
}
}
}
}
In the onPageFinished
method, we track the URL of the currently loaded page. Once the page finishes loading (e.g., Instagram’s login page), we can proceed to retrieve the cookies stored by the browser. Now, let’s see how to actually pull those cookies!
Retrieving Cookies Using CookieManager
Android provides the CookieManager
class, which allows us to manage and retrieve cookies stored in the WebView’s session. However, the cookies are returned as a plain string, with no structured format (like JSON or XML). So, we need to split and process the string ourselves. Here’s a function to help us do just that:
fun getCookie(url: String, cookieName: String): String? {
// Initialize the CookieManager instance
val cookieManager = CookieManager.getInstance()
// Retrieve all cookies as a single string
val cookies = cookieManager.getCookie(url)
// If there are cookies, split them by ";" to separate each cookie
cookies?.let {
val cookieArray = it.split(";").toTypedArray()
// Loop through each cookie and split by "=" to get name-value pairs
for (cookie in cookieArray) {
val cookiePair = cookie.split("=").toTypedArray()
// Check if the current cookie name matches the one we're looking for
if (cookiePair[0].trim() == cookieName) {
// Return the value of the cookie
return cookiePair[1]
}
}
}
// Return null if the cookie isn't found
return null
}
This function, getCookie
, takes the URL and the specific cookie name you’re interested in as parameters. It retrieves the cookies for the given URL and parses them into a more usable format. If it finds the cookie you want, it returns the value of that cookie, otherwise, it returns null.
Important: Make sure you call this function after the page finishes loading, as cookies may not be available before that point.
Attaching the WebViewClient to the WebView
Now that we have our custom WebViewClient and our cookie retrieval function, we need to connect them. This ensures that as the user navigates through the WebView, we can capture the necessary cookies after each page load. Here’s how we attach the client and load the URL:
binding.webView.webViewClient = MyWebViewClient()
// Load the Instagram login page
binding.webView.loadUrl("https://www.instagram.com/accounts/login/")
In this setup, every time the WebView navigates to a new page, onPageFinished
is called, allowing us to retrieve the cookies for the current page.
Enhancing Security with Cookie Management
When dealing with cookies, especially those containing sensitive data (like session tokens), it’s essential to handle them securely. Avoid storing these cookies in plain text or insecurely passing them around. Always encrypt sensitive information when necessary and avoid exposing user data.
Pro Tip: Use secure storage mechanisms (like Android’s SharedPreferences
with encryption) to store any sensitive data retrieved from cookies.
Conclusion: Managing Cookies in Android WebView
And there you have it! By using Android’s WebView
and CookieManager
, you can efficiently manage and retrieve cookies during your app’s WebView sessions. Whether you’re maintaining a session between web and app, or simply accessing user preferences, knowing how to handle cookies is a valuable skill in mobile development.
Thanks for following along! I hope this guide helps you streamline cookie management in your Android apps. Happy coding! 😊
Source: CookieManager Documentation